Developing a Memo App with Tkinter (1) - Running a 'Hello World' Program
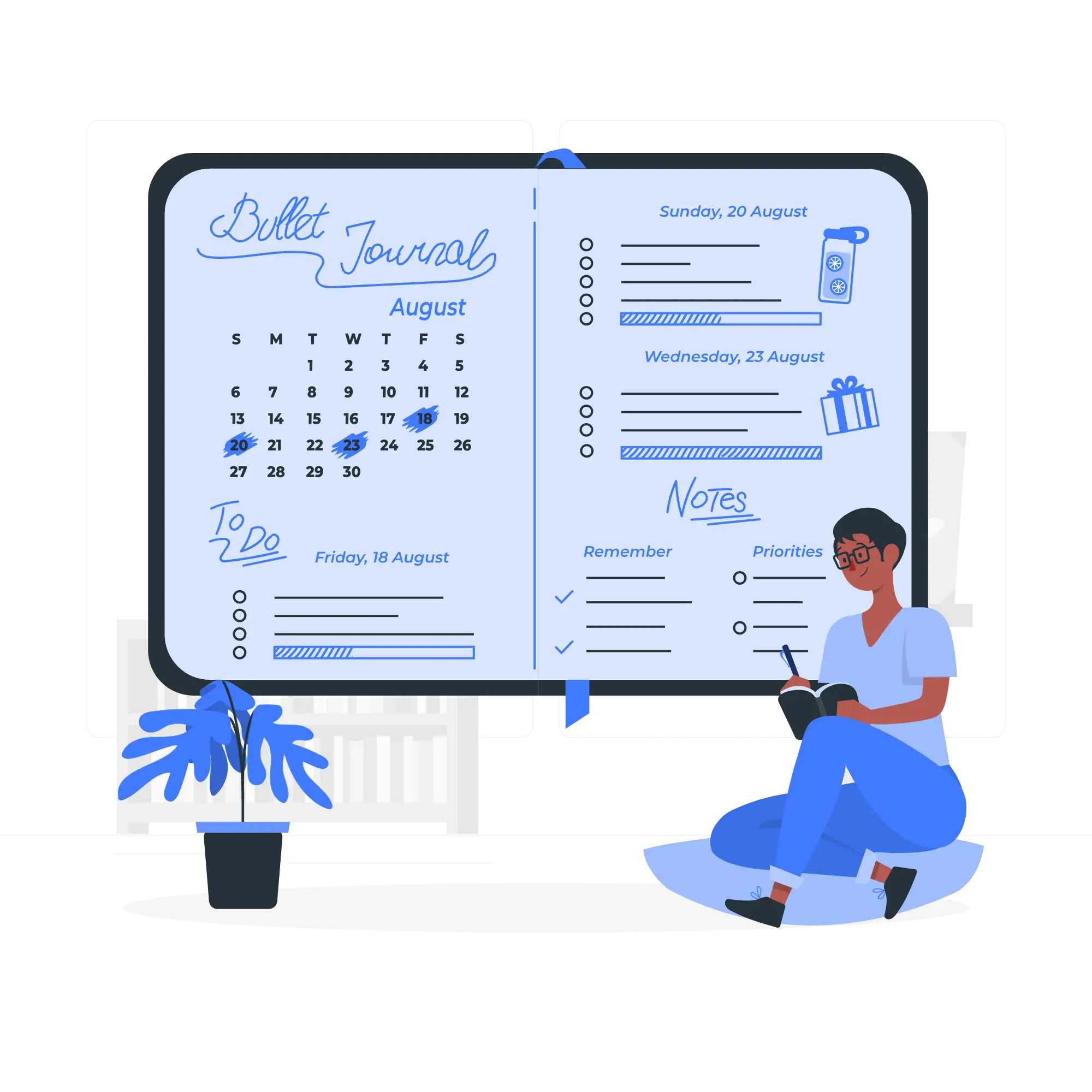
In this series, we’ll be leveraging Tkinter, Python’s built-in GUI library, to create a memo app.
Getting Started with the “Hello World” Program
Let’s begin by exploring how to use Tkinter with the following “Hello World” program outlined in its official documentation.
from tkinter import *
from tkinter import ttk
root = Tk()
frm = ttk.Frame(root, padding=10)
frm.grid()
ttk.Label(frm, text="Hello World!").grid(column=0, row=0)
ttk.Button(frm, text="Quit", command=root.destroy).grid(column=1, row=0)
root.mainloop()
Upon execution, this script will display a window like the one shown below.

Clicking the “Quit” button will close the window.
Understanding the Code
Let’s look at the details of the Hello World program.
1. Importing Libraries
from tkinter import *
from tkinter import ttk
Here, we import objects from tkinter
. Importing all objects with a wildcard (*
) is generally discouraged, so we’ll fix it later.
2. Creating the Root Window
root = Tk()
We start by creating the root window, which will house various components like buttons and text boxes.
In tkinter, these components are referred to as widgets.
3. Setting Up the Frame
frm = ttk.Frame(root, padding=10)
frm.grid()
Next, a frame widget is added to the root window. The frm.grid()
call places the frame frm
within the root window.
4. Placing a Label
ttk.Label(frm, text="Hello World!").grid(column=0, row=0)
A label, which displays text, is then placed within the frame (frm
).
We set the label’s text to “Hello World!” and position it at column=0, row=0
.
5. Adding a Button
ttk.Button(frm, text="Quit", command=root.destroy).grid(column=1, row=0)
A button is placed next to the label in the frame (frm
).
Its text is set to “Quit”, and it is programmed to execute root.destroy
when clicked, closing the root window.
- The label and the button share the same row (
row
), but the button is positioned right next to the label (column
). - Executing the
root.destroy
function will terminate the root window (root
).
6. Executing the Loop
root.mainloop()
Finally, we run the main loop of the root window (root
), which keeps the window visible and responsive until stopped. To stop the program, you can press Ctrl+C
in the console where the program was launched.
Conclusion
In this tutorial, we familiarized ourselves with Tkinter by setting up and running a simple “Hello World” program from the official documentation.
Next time, we’ll create an app with a text box and add functionality to save a memo to a text file.