Building a Discord Chatbot with Python (4) - Receiving Messages with Your Chatbot
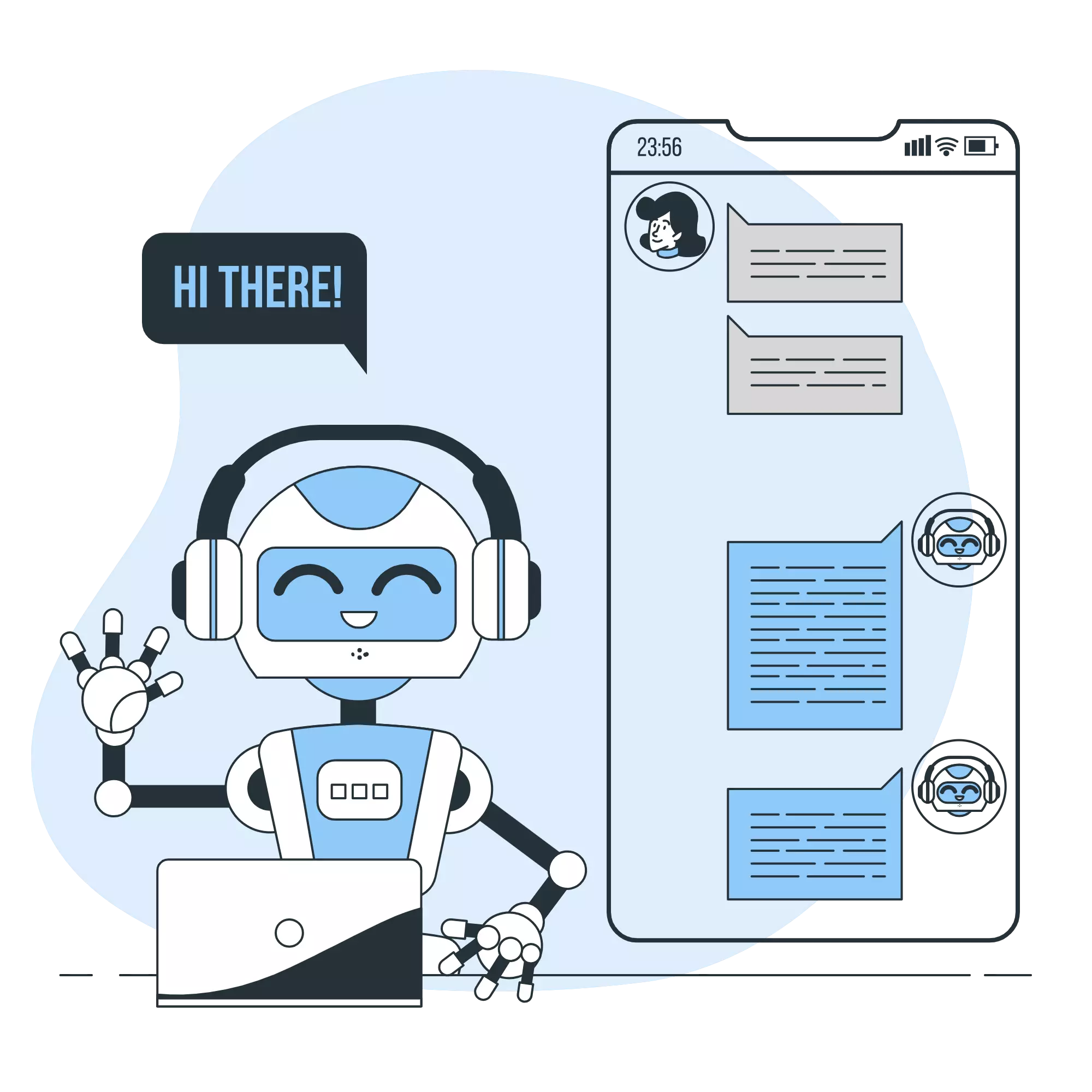
In this article, we’ll finally dive into creating a chatbot in Python.
Firstly, ensure that your working directory, discord-chatbot
, contains at least the following two components from our previous sessions:
.venv
directory: This is for a virtual environment.
.discord_token
file: This file contains a Discord token.
1. Activating the Virtual Environment
-
Move to the
discord-chatbot
directory and activate the virtual environment:cd discord-chatbot source .venv/bin/activate
-
Ensure
discord.py
is installed:pip list
2. Connecting Your Chatbot to Discord
2.1. Script
To begin, we’ll build a basic chatbot that only connects to Discord.
Create a file named chatbot.py
and write the following code:
import discord
with open('.discord_token') as f:
token = f.read().strip()
intents = discord.Intents.default()
client = discord.Client(intents=intents)
client.run(token)
Then execute the script.
python chatbot.py
If everything goes well, the console should display logs indicating a successful connection to Discord, like the one below:
2023-08-18 23:39:36 INFO discord.client logging in using static token
2023-08-18 23:39:37 INFO discord.gateway Shard ID None has connected to Gateway (Session ID: xxx).
Terminate the bot using Ctrl+C
.
2.2. Code Breakdown
Let’s dissect the script step-by-step:
Step 1
import discord
Here, we’re importing the discord.py
library.
Step 2
with open('.discord_token') as f:
token = f.read().strip()
This block reads the token stored in the .discord_token
file and assigns it to the token
variable.
The strip
function ensures any trailing newline characters are removed.
Step 3
intents = discord.Intents.default()
discord.Intents
defines the types of events a chatbot will subscribe to.
Here, we’re using the default settings.
Step 4
client = discord.Client(intents=intents)
This line initializes the Discord client, which acts as the backbone of our chatbot.
Step 5
client.run(token)
Lastly, we’re using the token to launch the client, thus activating our chatbot.
3. Enhancing Your Chatbot’s Functionality
3.1 Create a Custom Class by Inheriting from discord.Client
Let’s further enhance our chatbot by adding more features.
While discord.py
offers a myriad of methods for adding features, in this series, we’ll focus on creating a custom class derived from the discord.Client
class and making modifications to it.
3.2 The on_ready
and on_message
Methods
Update chatbot.py
as shown below:
import discord
class MyClient(discord.Client):
async def on_ready(self):
"""Triggered when connecting to Discord."""
print(f'Connected as {self.user}.')
async def on_message(self, message: discord.Message):
"""Triggered when receiving a message."""
print(f'Received a message from {message.author}: {message.content}')
with open('.discord_token') as f:
token = f.read().strip()
intents = discord.Intents.default()
client = MyClient(intents=intents)
client.run(token)
3.3 Code Breakdown
- We’ve defined a class named
MyClient
that inherits from thediscord.Client
.
Within this class, we’ve overridden two functions:on_ready
andon_message
. - Their respective purposes are:
on_ready
: Triggered when connecting to Discord.on_message
: Triggered when receiving a message.
- Both functions are prefaced with the keyword
async
, denoting them as coroutine functions - specialized functions designed for asynchronous operations. We’ll delve deeper into this in upcoming sessions. self.user
holds the bot’s username.- The
message
argument inon_message
receives data of the type discord.Message.
Here,message.author
denotes the sender andmessage.content
contains the message text.
Strings like f'Connected as {self.user}.'
are called formatted string literals or f-strings
.
Introduced in Python 3.6, they allow the inclusion of embedded expressions inside string literals.
(Ref: Formatted string literals)
It offers a concise way to embed values into strings, so we’ll use them a lot throughout this series.
3.4 Let’s Run It
-
Let’s give it a go:
python chatbot.py
-
Upon connection, you’ll see a message on the console, something akin to:
Successfully connected as xxx.
. -
If you type a message in the chat, the message like
Received a message from xxx:
will appear in your console. However, The message content isn’t being displayed!
3.5 Adjusting Intents Settings to Receive Message Content
Let’s refer to the documentation. There, you might stumble upon:
If
Intents.message_content
is not enabled this will always be an empty string unless the bot is mentioned or the message is a direct message.
Ah, we need to correctly set discord.Intents
. To fetch the content of a message, it’s imperative to set the intents.message_content
value to True
.
intents.message_content = True
Add this to the code and re-run script!
You’ll now see messages like: Received a message from xxx: yyy
.
4. Conclusion
In this article, we successfully connected our chatbot to Discord and configured it to receive user messages.
In the next article, we’ll set up the bot to respond directly to these messages in the chat.
Final Code
import discord
class MyClient(discord.Client):
async def on_ready(self):
"""Triggered when connecting to Discord."""
print(f'Connected as {self.user}.')
async def on_message(self, message: discord.Message):
"""Triggered when receiving a message."""
print(f'Received a message from {message.author}: {message.content}')
with open('.discord_token') as f:
token = f.read().strip()
intents = discord.Intents.default()
intents.message_content = True
client = MyClient(intents=intents)
client.run(token)