Exploring LangChain's Quickstart (3) - Utilizing Conversation History
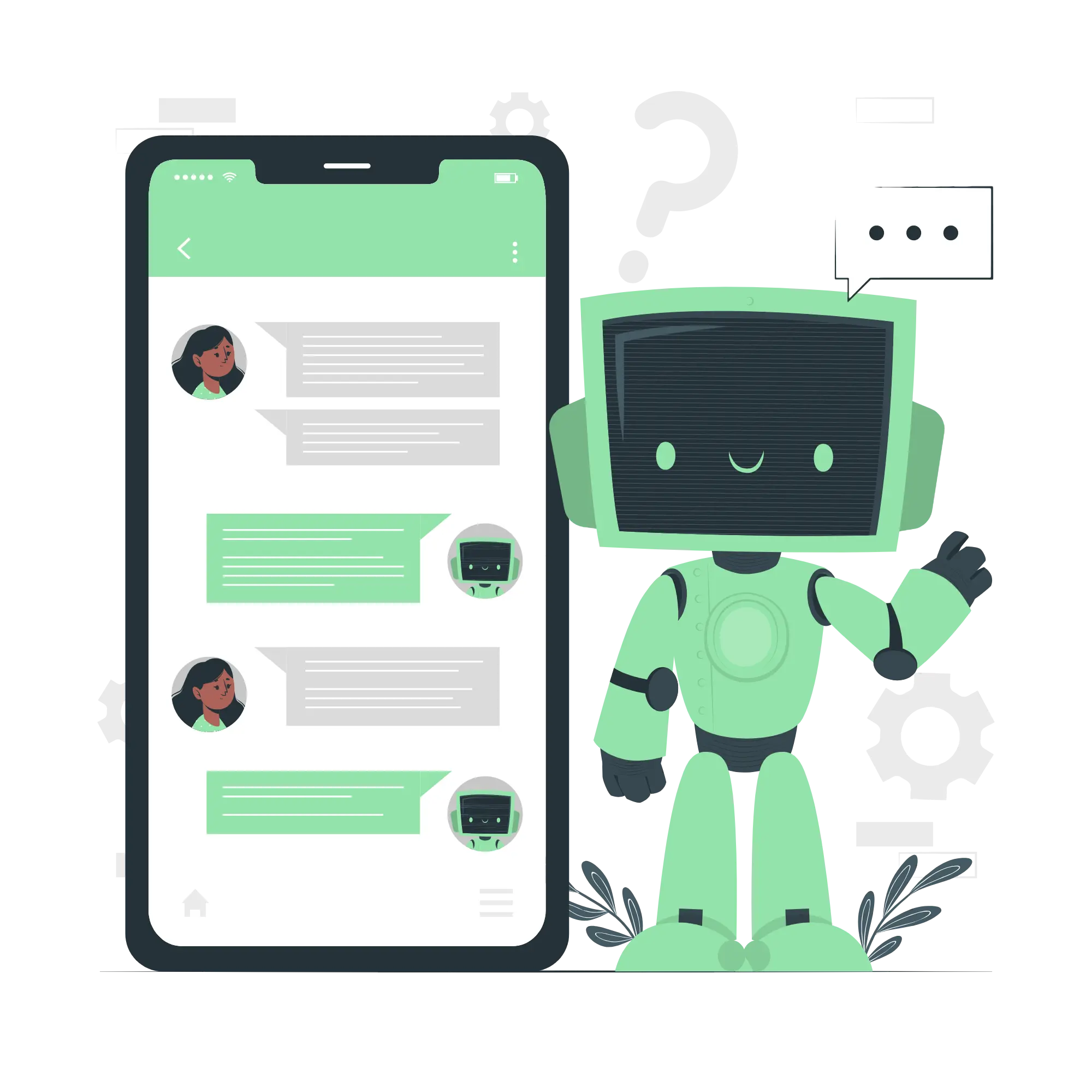
In this post, we continue our journey through LangChain’s Quickstart guide, exploring how to enhance your chains by integrating conversation history.
Recap of Our Progress
Here’s what we’ve set up so far:
retriever
: Retrives a list of relevant documents based on the input text.document_chain
: Generates LLM responses using the user’s questions and the list of documents.create_retrieval_chain
: Combinesretriever
anddocument_chain
to answer queries by referencing documents.
import os
from langchain_openai import ChatOpenAI, OpenAIEmbeddings
from langchain_community.document_loaders import WebBaseLoader
from langchain_community.vectorstores import FAISS
from langchain_text_splitters import RecursiveCharacterTextSplitter
# Setting up the API key in environment variable
with open('.openai') as f:
os.environ['OPENAI_API_KEY'] = f.read().strip()
# Loading the LLM
llm = ChatOpenAI()
# Loading web page content
loader = WebBaseLoader("https://python.langchain.com/docs/get_started/introduction")
docs = loader.load()
# Loading embeddings
embeddings = OpenAIEmbeddings()
# Splitting documents
text_splitter = RecursiveCharacterTextSplitter()
documents = text_splitter.split_documents(docs)
# Vectorizing documents and creating a vector store
vector = FAISS.from_documents(documents, embeddings)
# Creating a retriever
retriever = vector.as_retriever()
7. Enhancing the Retrieval Chain with Conversation History
To incorporate conversation history, we make the following adjustments:
- Modify the
retriever
to reference conversation history. - Modify the
document_chain
to also reference conversation history.
7.1. Adjusting the retriever
to Reference Conversation History
We create a retriever that extracts relevant documents by considering conversation history through the following steps:
- Use the conversation history to generate a search query with the LLM.
- Use the query to retrieve appropriate documents from the vector store.
7.1.1. Generating a Search Query from Conversation History
First, we set up a prompt template that utilizes conversation history:
from langchain_core.prompts import ChatPromptTemplate, MessagesPlaceholder
template = ChatPromptTemplate.from_messages([
MessagesPlaceholder(variable_name="chat_history"),
("user", "{input}"),
("user", "Please generate a search query relevant to the above conversation.")
])
You input the chat history as follows:
from langchain_core.messages import HumanMessage, AIMessage
query_chain = template | llm
chat_history = [
HumanMessage(content="How do I install LangChain?"),
AIMessage(content="You can install LangChain using pip install langchain.")
]
search_query = query_chain.invoke({
"chat_history": chat_history,
"input": "Can you explain more about it?"
})
search_query
-
Result:
AIMessage(content='"LangChain installation guide"', response_metadata={'token_usage': {'completion_tokens': 6, 'prompt_tokens': 55, 'total_tokens': 61}, 'model_name': 'gpt-3.5-turbo', 'system_fingerprint': None, 'finish_reason': 'stop', 'logprobs': None}, id='run-xxxxx')
7.1.2. Fetching Related Documents with the Generated Search Query
Next, we use the generated search query to retrieve related documents, leveraging the previously created retriever
.
retriever.invoke(search_query.content)
-
Result:
[Document(page_content='Introduction | 🦜️🔗 LangChain', metadata={'source': 'https://python.langchain.com/docs/get_started/introduction', 'title': 'Introduction | 🦜️🔗 LangChain', 'description': 'LangChain is a framework for developing applications powered by large language models (LLMs).', 'language': 'en'}), Document(page_content="Skip to main contentLangChain v0.2 is coming soon! Preview the new docs here.ComponentsIntegrationsGuidesAPI ReferenceMorePeopleVersioningContributingTemplatesCookbooksTutorialsYouTube🦜️🔗LangSmithLangSmith DocsLangServe GitHubTemplates GitHubTemplates HubLangChain HubJS/TS Docs💬SearchGet startedIntroductionQuickstartInstallationUse casesQ&A with RAGExtracting structured outputChatbotsTool use and agentsQuery analysisQ&A over SQL + CSVMoreExpression LanguageGet startedRunnable interfacePrimitivesAdvantages of LCELStreamingAdd message history (memory)MoreEcosystem🦜🛠️ LangSmith🦜🕸️LangGraph🦜️🏓 LangServeSecurityGet startedOn this pageIntroductionLangChain is a framework for developing applications powered by large language models (LLMs).LangChain simplifies every stage of the LLM application lifecycle:Development: Build your applications using LangChain's open-source building blocks and components. Hit the ground running using third-party integrations and Templates.Productionization: Use LangSmith to inspect, monitor and evaluate your chains, so that you can continuously optimize and deploy with confidence.Deployment: Turn any chain into an API with LangServe.Concretely, the framework consists of the following open-source libraries:langchain-core: Base abstractions and LangChain Expression Language.langchain-community: Third party integrations.Partner packages (e.g. langchain-openai, langchain-anthropic, etc.): Some integrations have been further split into their own lightweight packages that only depend on langchain-core.langchain: Chains, agents, and retrieval strategies that make up an application's cognitive architecture.langgraph: Build robust and stateful multi-actor applications with LLMs by modeling steps as edges and nodes in a graph.langserve: Deploy LangChain chains as REST APIs.The broader ecosystem includes:LangSmith: A developer platform that lets you debug, test, evaluate, and monitor LLM applications and seamlessly integrates with LangChain.Get started\u200bWe recommend following our Quickstart guide to familiarize yourself with the framework by building your first LangChain application.See here for instructions on how to install LangChain, set up your environment, and start building.noteThese docs focus on the Python LangChain library. Head here for docs on the JavaScript LangChain library.Use cases\u200bIf you're looking to build something specific or are more of a hands-on learner, check out our use-cases.", metadata={'source': 'https://python.langchain.com/docs/get_started/introduction', 'title': 'Introduction | 🦜️🔗 LangChain', 'description': 'LangChain is a framework for developing applications powered by large language models (LLMs).', 'language': 'en'}), Document(page_content="They're walkthroughs and techniques for common end-to-end tasks, such as:Question answering with RAGExtracting structured outputChatbotsand more!Expression Language\u200bLangChain Expression Language (LCEL) is the foundation of many of LangChain's components, and is a declarative way to compose chains. LCEL was designed from day 1 to support putting prototypes in production, with no code changes, from the simplest “prompt + LLM” chain to the most complex chains.Get started: LCEL and its benefitsRunnable interface: The standard interface for LCEL objectsPrimitives: More on the primitives LCEL includesand more!Ecosystem\u200b🦜🛠️ LangSmith\u200bTrace and evaluate your language model applications and intelligent agents to help you move from prototype to production.🦜🕸️ LangGraph\u200bBuild stateful, multi-actor applications with LLMs, built on top of (and intended to be used with) LangChain primitives.🦜🏓 LangServe\u200bDeploy LangChain runnables and chains as REST APIs.Security\u200bRead up on our Security best practices to make sure you're developing safely with LangChain.Additional resources\u200bComponents\u200bLangChain provides standard, extendable interfaces and integrations for many different components, including:Integrations\u200bLangChain is part of a rich ecosystem of tools that integrate with our framework and build on top of it. Check out our growing list of integrations.Guides\u200bBest practices for developing with LangChain.API reference\u200bHead to the reference section for full documentation of all classes and methods in the LangChain and LangChain Experimental Python packages.Contributing\u200bCheck out the developer's guide for guidelines on contributing and help getting your dev environment set up.Help us out by providing feedback on this documentation page:NextIntroductionGet startedUse casesExpression LanguageEcosystem🦜🛠️ LangSmith🦜🕸️ LangGraph🦜🏓 LangServeSecurityAdditional resourcesComponentsIntegrationsGuidesAPI referenceContributingCommunityDiscordTwitterGitHubPythonJS/TSMoreHomepageBlogYouTubeCopyright © 2024 LangChain, Inc.", metadata={'source': 'https://python.langchain.com/docs/get_started/introduction', 'title': 'Introduction | 🦜️🔗 LangChain', 'description': 'LangChain is a framework for developing applications powered by large language models (LLMs).', 'language': 'en'})]
7.1.3. Automating with create_history_aware_retriever
Using create_history_aware_retriever
, we can automate the process described above to create a retriever that accounts for conversation history.
from langchain.chains import create_history_aware_retriever
from langchain_core.prompts import MessagesPlaceholder
template = ChatPromptTemplate.from_messages([
MessagesPlaceholder(variable_name="chat_history"),
("user", "{input}"),
("user", "Generate a search query relevant to the conversation above.")
])
retriever_chain = create_history_aware_retriever(llm, retriever, template)
-
Example:
chat_history = [ HumanMessage(content="How do I install LangChain?"), AIMessage(content="You can install LangChain using pip install langchain.") ] context = retriever_chain.invoke({ "chat_history": chat_history, "input": "Can you provide more details?" }) context
-
Result:
[Document(page_content="Skip to main contentLangChain v0.2 is coming soon! Preview the new docs here.ComponentsIntegrationsGuidesAPI ReferenceMorePeopleVersioningContributingTemplatesCookbooksTutorialsYouTube🦜️🔗LangSmithLangSmith DocsLangServe GitHubTemplates GitHubTemplates HubLangChain HubJS/TS Docs💬SearchGet startedIntroductionQuickstartInstallationUse casesQ&A with RAGExtracting structured outputChatbotsTool use and agentsQuery analysisQ&A over SQL + CSVMoreExpression LanguageGet startedRunnable interfacePrimitivesAdvantages of LCELStreamingAdd message history (memory)MoreEcosystem🦜🛠️ LangSmith🦜🕸️LangGraph🦜️🏓 LangServeSecurityGet startedOn this pageIntroductionLangChain is a framework for developing applications powered by large language models (LLMs).LangChain simplifies every stage of the LLM application lifecycle:Development: Build your applications using LangChain's open-source building blocks and components. Hit the ground running using third-party integrations and Templates.Productionization: Use LangSmith to inspect, monitor and evaluate your chains, so that you can continuously optimize and deploy with confidence.Deployment: Turn any chain into an API with LangServe.Concretely, the framework consists of the following open-source libraries:langchain-core: Base abstractions and LangChain Expression Language.langchain-community: Third party integrations.Partner packages (e.g. langchain-openai, langchain-anthropic, etc.): Some integrations have been further split into their own lightweight packages that only depend on langchain-core.langchain: Chains, agents, and retrieval strategies that make up an application's cognitive architecture.langgraph: Build robust and stateful multi-actor applications with LLMs by modeling steps as edges and nodes in a graph.langserve: Deploy LangChain chains as REST APIs.The broader ecosystem includes:LangSmith: A developer platform that lets you debug, test, evaluate, and monitor LLM applications and seamlessly integrates with LangChain.Get started\u200bWe recommend following our Quickstart guide to familiarize yourself with the framework by building your first LangChain application.See here for instructions on how to install LangChain, set up your environment, and start building.noteThese docs focus on the Python LangChain library. Head here for docs on the JavaScript LangChain library.Use cases\u200bIf you're looking to build something specific or are more of a hands-on learner, check out our use-cases.", metadata={'source': 'https://python.langchain.com/docs/get_started/introduction', 'title': 'Introduction | 🦜️🔗 LangChain', 'description': 'LangChain is a framework for developing applications powered by large language models (LLMs).', 'language': 'en'}), Document(page_content='Introduction | 🦜️🔗 LangChain', metadata={'source': 'https://python.langchain.com/docs/get_started/introduction', 'title': 'Introduction | 🦜️🔗 LangChain', 'description': 'LangChain is a framework for developing applications powered by large language models (LLMs).', 'language': 'en'}), Document(page_content="They're walkthroughs and techniques for common end-to-end tasks, such as:Question answering with RAGExtracting structured outputChatbotsand more!Expression Language\u200bLangChain Expression Language (LCEL) is the foundation of many of LangChain's components, and is a declarative way to compose chains. LCEL was designed from day 1 to support putting prototypes in production, with no code changes, from the simplest “prompt + LLM” chain to the most complex chains.Get started: LCEL and its benefitsRunnable interface: The standard interface for LCEL objectsPrimitives: More on the primitives LCEL includesand more!Ecosystem\u200b🦜🛠️ LangSmith\u200bTrace and evaluate your language model applications and intelligent agents to help you move from prototype to production.🦜🕸️ LangGraph\u200bBuild stateful, multi-actor applications with LLMs, built on top of (and intended to be used with) LangChain primitives.🦜🏓 LangServe\u200bDeploy LangChain runnables and chains as REST APIs.Security\u200bRead up on our Security best practices to make sure you're developing safely with LangChain.Additional resources\u200bComponents\u200bLangChain provides standard, extendable interfaces and integrations for many different components, including:Integrations\u200bLangChain is part of a rich ecosystem of tools that integrate with our framework and build on top of it. Check out our growing list of integrations.Guides\u200bBest practices for developing with LangChain.API reference\u200bHead to the reference section for full documentation of all classes and methods in the LangChain and LangChain Experimental Python packages.Contributing\u200bCheck out the developer's guide for guidelines on contributing and help getting your dev environment set up.Help us out by providing feedback on this documentation page:NextIntroductionGet startedUse casesExpression LanguageEcosystem🦜🛠️ LangSmith🦜🕸️ LangGraph🦜🏓 LangServeSecurityAdditional resourcesComponentsIntegrationsGuidesAPI referenceContributingCommunityDiscordTwitterGitHubPythonJS/TSMoreHomepageBlogYouTubeCopyright © 2024 LangChain, Inc.", metadata={'source': 'https://python.langchain.com/docs/get_started/introduction', 'title': 'Introduction | 🦜️🔗 LangChain', 'description': 'LangChain is a framework for developing applications powered by large language models (LLMs).', 'language': 'en'})]
-
7.2. Adjusting the document_chain
to Reference Conversation History
We now modify the document_chain
, which generates LLM responses based on user input and document list, to reference conversation history.
This involves updating the template as follows:
from langchain.chains.combine_documents import create_stuff_documents_chain
template = ChatPromptTemplate.from_messages([
("system", "Please answer the user's question based on the following context:\n\n{context}"),
MessagesPlaceholder(variable_name="chat_history"),
("user", "{input}"),
])
document_chain = create_stuff_documents_chain(llm, template)
-
Example:
chat_history = [ HumanMessage(content="How do I install LangChain?"), AIMessage(content="You can install LangChain using pip install langchain.") ] document_chain.invoke({ "context": context, "chat_history": chat_history, "input": "Can you provide more details?" })
-
Result:
'Certainly! To install LangChain, you can follow these steps:\n\n1. Make sure you have Python installed on your system.\n2. Open your command line interface (CLI).\n3. Use the following command to install LangChain via pip:\n\n```\npip install langchain\n```\n\n4. Once the installation is complete, you can start using LangChain to develop applications powered by large language models.\n\nIf you encounter any issues during the installation process, feel free to ask for further assistance.'
-
7.3. Generating Responses by Referencing Conversation History and Documents
Finally, using the retriever_chain
and document_chain
, we create a chain that takes conversation history and documents as input to generate LLM responses. We use create_retrieval_chain
for this purpose.
from langchain.chains import create_retrieval_chain
retrieval_chain = create_retrieval_chain(retriever_chain, document_chain)
Here’s how you can generate a response using conversation history and user input:
chat_history = [
HumanMessage(content="How do I install LangChain?"),
AIMessage(content="You can install LangChain using pip install langchain.")
]
response = retrieval_chain.invoke({
"chat_history": chat_history,
"input": "Can you explain how to use it?"
})
print(response["answer"])
-
Result:
To use LangChain, you can follow these steps: 1. Install LangChain using pip install langchain. 2. Set up your environment and import the necessary modules. 3. Follow the Quickstart guide provided in the LangChain documentation to build your first LangChain application. 4. Familiarize yourself with the LangChain Expression Language (LCEL) to compose chains declaratively. 5. Utilize the LangSmith platform to debug, test, evaluate, and monitor your LLM applications seamlessly. 6. Deploy your LangChain chains as REST APIs using LangServe. By following these steps and exploring the documentation further, you can effectively use LangChain to develop applications powered by large language models.