How to Skip Cell Execution in Jupyter Notebook
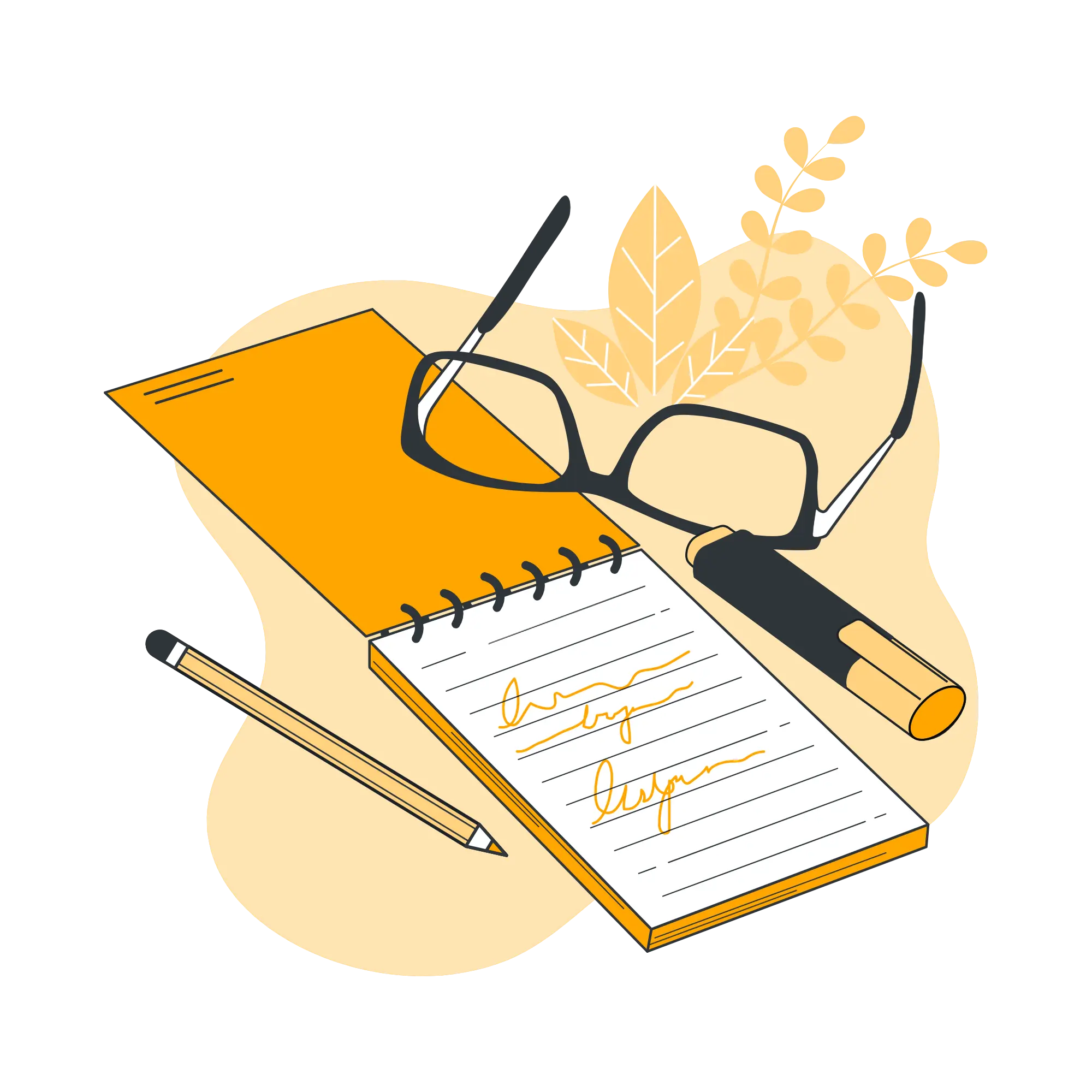
Contents
In this article, I’ll show you how to skip the execution of cells in Jupyter Notebook based on certain conditions.
The methods described here are applicable to Google Colaboratory and Kaggle Notebooks.
How to Skip a Cell Execution
To skip cell execution, create a custom magic command as follows:
-
First, create a
skip
magic command that does nothing.from IPython.core.magic import register_cell_magic @register_cell_magic def skip(line, cell): return
-
To skip a cell, simply add
%%skip
at the top line of the cell.%%skip <code>
How to Conditionally Skip a Cell Execution
Next, let’s explore how to skip cell execution based on a condition.
-
Create a
skip_if
magic command.from IPython.core.magic import register_cell_magic from IPython import get_ipython @register_cell_magic def skip_if(line, cell): if eval(line): return get_ipython().run_cell(cell)
-
To use it, add
%%skip_if <condition>
at the top of the cell.%%skip_if <condition> <code>
How to Skip All Subsequent Cells Based on a Condition
Finally, I will explain how to skip all subsequent cells based on a condition.
-
Modify the
run_cell
function as follows:from IPython import get_ipython SKIP_CONDITION = <condition> # Save the original run_cell run_cell_org = get_ipython().run_cell # Create a custom run_cell that skips execution based on the condition def custom_run_cell(*args, **kwargs): if SKIP_CONDITION: if 'raw_cell' in kwargs: kwargs['raw_cell'] = "" else: args = list(args) args[0] = "" args = tuple(args) return run_cell_org(*args, **kwargs) # Replace the original run_cell get_ipython().run_cell = custom_run_cell
-
All subsequent cells will be skipped if the
<condition>
is true.