How to Get Notifications When Exceptions Occur in Jupyter Notebook
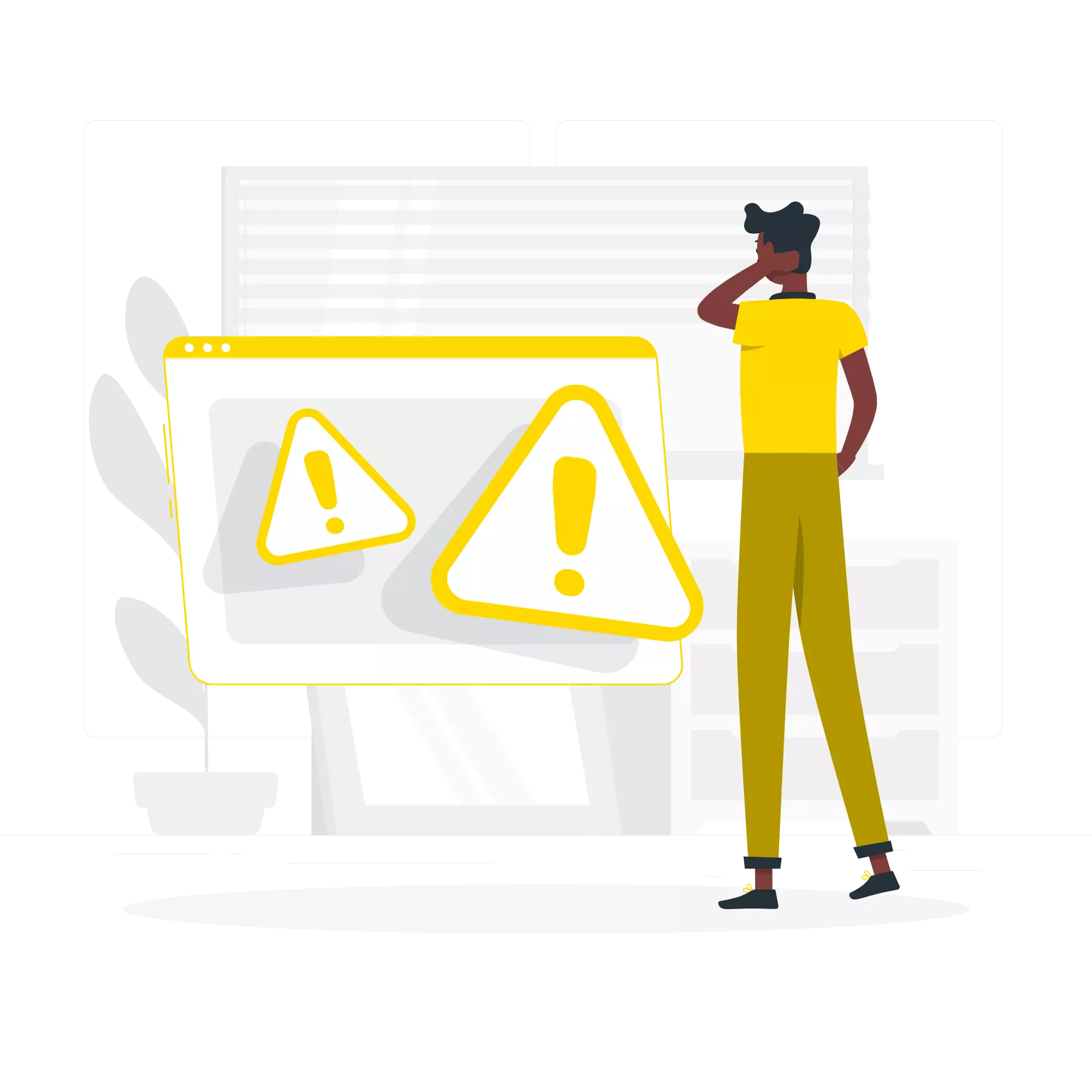
Have you ever been running a time-consuming code in Jupyter Notebook, like training a machine learning model, only to realize later that it had stopped due to an unexpected error?
In this article, I’ll introduce a method to automatically send notifications to platforms like Slack or Discord when such exceptions occur.
Creating a Notification Function
First, let’s prepare a function for sending notifications. If you’re using Slack or Discord, you can notify with the following functions:
-
Script for Slack Notification:
import requests def slack_notify(message, webhook_url): """Notify Slack.""" requests.post(webhook_url, json={"text": message})
-
Script for Discord Notification:
import requests def discord_notify(message, webhook_url): """Notify Discord.""" requests.post(webhook_url, json={"content": message})
Execute Script in Jupyter Notebook When an Exception Occurs
By using the set_custom_exc function, you can add additional processes during exceptions.
The code below is mostly based on this answer from stackoverflow.
from IPython import get_ipython
from IPython.core.ultratb import AutoFormattedTB
def jupyter_preprocess():
"""Function to run at the start of Jupyter Notebook."""
# ref. https://stackoverflow.com/a/40135960
itb = AutoFormattedTB(mode='Plain', tb_offset=1)
def custom_exc(shell, etype, evalue, tb, tb_offset=None):
shell.showtraceback((etype, evalue, tb), tb_offset=tb_offset)
stb = itb.structured_traceback(etype, evalue, tb)
sstb = itb.stb2text(stb)
# Write the code to be executed during an exception here
slack_notify("ERROR: An exception has occurred.", "<WEBHOOK URL>")
return sstb
get_ipython().set_custom_exc((Exception,), custom_exc)
By running the above jupyter_preprocess
function at the start, you’ll receive notifications when exceptions occur. As the code shows, you can also implement other processes besides notifications, like server shutdowns, and so on.